Initialize a Checkout Session
To initialize a Checkout session, a POST request to the checkout backend API endpoint is performed. This will return a public token that is used to render the Checkout iframe and a private id that is used to retrieve information about the Checkout session at a later step.
- Example Request
- Example Response
- Example Faulty Response
/*
POST /checkouts HTTP/1.1
Host: api.uat.walleydev.com // (Please note! Different hostname in production)
Authorization: Bearer bXlVc2VybmFtZTpmN2E1ODA4MGQzZTk0M2VmNWYyMTZlMDE...
Content-Type: application/json
*/
{
"storeId": 123,
"countryCode": "SE",
"reference": "123456789",
"redirectPageUri": "https://example.com/purchase-completed-confirmation-page",
"checkoutAbortedRedirectPageUri": "https://example.com/back-to-your-shop",
"merchantTermsUri": "https://example.com/merchant-purchase-terms",
"notificationUri": "https://example.com/my-notification-endpoint",
"validationUri": "https://example.com/my-validation-endpoint",
"callback": {
"callbackUri": "https://example.com/my-callback-endpoint",
"callbackTypes": ["ValidateOrder", "PaymentFailed"]
},
"profileName": "MyExampleProfile",
"settlementReference": "shop1",
"externalStoreId": "shop1",
"actionReference": "MyActionReference",
"cart": {
"items": [
{
"id": "10001",
"description": "A product description",
"unitPrice": 95.0,
"unitWeight": 0.3,
"quantity": 1,
"vat": 25.0,
"requiresElectronicId": true,
"sku": "a unique alphanumeric code for article identification"
},
{
"id": "10002",
"description": "Gift Card",
"type": "GiftCard",
"unitPrice": -100.0,
"quantity": 1,
"vat": 0.0
}
],
"shippingProperties": {
"height": 10,
"width": 20,
"isBulky": true
}
},
"fees": {
"shipping": {
"id": "shipping001",
"description": "Shipping cost (incl. VAT)",
"unitPrice": 59.0,
"vat": 25.0
}
},
"privateCustomerPrefill": {
"email": "test@walleypay.se",
"mobilePhoneNumber": "+46701234567",
"nationalIdentificationNumber": "19520101-1234",
"deliveryAddress": {
"firstName": "John",
"lastName": "Doe",
"coAddress": "c/o Jane Doe",
"address": "Sunny road 2",
"address2": "Another address line if needed",
"postalCode": "12345",
"city": "Big Town",
"countryCode": "SE"
}
},
"businessCustomerPrefill": {
"organizationNumber": "5562000116",
"buyer": {
"firstName": "John",
"lastName": "Doe",
"email": "test@walleypay.se",
"mobilePhoneNumber": "+46701234567"
},
"invoiceReference": "ref",
"invoiceTag": "tag",
"deliveryAddress": {
"companyName": "No 3 mining enterprise",
"coAddress": "c/o Jane Doe",
"address": "Rosenborgsgatan 4",
"address2": "Another address line if needed",
"postalCode": "16974",
"city": "Solna"
}
},
"businessCustomerPreset": {
"organizationNumber": "5562000116",
"buyer": {
"firstName": "John",
"lastName": "Doe",
"email": "test@walleypay.se",
"mobilePhoneNumber": "+46701234567"
},
"invoiceReference": "ref",
"invoiceTag": "tag",
"deliveryAddress": {
"companyName": "No 3 mining enterprise",
"coAddress": "c/o Jane Doe",
"address": "Rosenborgsgatan 4",
"address2": "Another address line if needed",
"postalCode": "16974",
"city": "Solna"
}
},
"customFields": [
{
"id": "group1",
"metadata": {
"groupMeta": "content"
},
"fields": [
{
"id": "newsConsent",
"name": "I agree to receive marketing communications",
"type": "Checkbox",
"value": true,
"metadata": {
"field1Meta": "field-newsletter-consent"
},
"localizations": {
"fi-FI": {
"name": "Hyväksyn markkinointiviestinnän vastaanottamisen",
"value": false,
"metadata": {
"field1Meta": "fi-field-newsletter-consent"
}
}
}
},
{
"id": "comments",
"name": "Any additional comments?",
"type": "Text"
}
]
}
],
"metadata": {
"administrator": "John Doe",
"someOtherData": [1, { "myObj": { "key": 1 } }]
}
}
{
"id": "91714012-6ae9-4780-a927-fe459bc95bf6",
"data": {
"privateId": "1eec44b5-66d3-4058-a31f-3444229fb727",
"publicToken": "public-SE-7f1b3d2a2a73d348dfbd17d3965ff1458c249f84c695eac1",
"expiresAt": "2017-12-07T07:16:49.8098107+00:00",
"paymentUri": "https://checkout.uat.walleydev.com/p/npBc87EFB",
"paymentQrCodeUri": "https://api.uat.walleydev.com/checkouts/paylinks/npBc87EFB/qrcode"
},
"error": null
}
// Example of Initialize Checkout response where an invalid StoreId was provided
{
"id": "1da39a61-d3e1-4da5-a1a0-7a315ea66d2f",
"data": null,
"error": {
"code": 400,
"message": "Bad or faulty request. Please examine the errors property for details.",
"errors": [
{
"reason": "Store_Invalid",
"message": "Invalid store id or country code."
}
]
}
}
Request
Request headers
Header | Required | Explanation |
---|---|---|
Authorization | Yes | Instructions on how to generate the authorization header value can be found here. |
Request Body Properties
Property | Required | Explanation |
---|---|---|
countryCode | Yes | The country code to use. SE , NO , FI , DK , DE and EU is supported. |
storeId | No | Received from Walley Merchant Services. The store ID is only required in the request when integrating multiple stores with Walley Checkout. |
externalStoreId | No | Your own id of the store. This is sent to Voyado if you use them as a CRM system. It has a 50-character limit. |
reference | No | This is a reference to the order, like an order ID. The reference provided here will be shown to the customer on the invoice or receipt for the purchase. It has a 50-character limit. ** See special note below regarding Vipps |
salesSegment | No | If you have at most one B2C and/or B2B store in the selected country, then you can use this property to select the store instead of providing a store ID. The valid values are B2C and B2B . |
settlementReference | No * | Set this if you would like to group your settlement reports based on this value. Can for example be used when you have several shops connected to one storeId and want purchases to be grouped by individual shops. It has a 50-character limit. * To use this feature you must contact Merchant Services to setup Settlement Settings on this specific Store ID |
actionReference | No | A reference to this specific checkout session. This will appear as a data property on the settlement report if the order is auto captured. If you manually capture the order you should set the actionReference on the capture call. Maximum 255 characters. |
redirectPageUri | No * | If set, the browser will redirect to this page once the purchase has been completed. Used to display a thank-you-page for the end user. Hereafter referred to as the purchase confirmation page. * Required if the Store is an InStore type. |
checkoutAbortedRedirectPageUri | No | If set and rendering a Pay Link, a go back button will appear for the customer and if clicked, the checkout session will be deleted and the browser will redirect to this page. |
merchantTermsUri | Yes | The page to which the Checkout will include a link for customers that wish to view the merchant terms for purchase. |
notificationUri | Yes | The endpoint to be called whenever an event has occurred in the Walley Checkout that might be of interest. For example, this callback is typically used to create an order in the merchant's system once a purchase has been completed. Use HTTPS here for security reasons. |
validationUri | No | Specify this uri when you want us to make a GET backend call to validate the articles during purchase. Use HTTPS for security reasons. This method is replaced with the more generic callback functionality described below, but may still be used. Please use only one of the validation methods |
callback | No | Specify this object when you want us to make a POST backend call for any of the specified callback types. See description below for more information. |
profileName | No | A name that referes to a specific settings profile. The profiles are setup by Merchant Services, please contact them for more information help@walley.se |
cart | Yes | The initial Cart object with items to purchase. Details below |
fees | No | Shipping fee. Details below |
privateCustomerPrefill | No | Prefilled private customer information. Details below |
businessCustomerPrefill | No | Prefilled business customer information. Details below |
businessCustomerPreset | No | Preset business customer information. Details below |
customFields | No | Custom inputs to be rendered inside the checkout. Details below |
appRedirectPageUri | No | When checkout redirects to a partner app, the appRedirectPageUri is passed along (if supported) to ensure that the user is correctly returned to your app upon completion. It is specifically intended for use when the checkout is being rendered within an app and not on a website. This field should contain the URI of your app, which typically follows the format yourapp://. |
**
Special note regarding VippsFor Vipps purchases with settlements directly from Vipps using your own agreement, the reference
field can be used to match the purchase with the settlement, if a reference
is not provided the orderId
will be used instead.
The reference
or orderId
field is concatenated with a postfix of a dash -
and 9 characters. For example a reference of 12345
might result in a settlement reference of 12345-An1c8BAlI
.
This is also returned in the externalPaymentReference
field in the response when retrieving information about the checkout.
Callback object
The callback
object allows you to handle certain events within the Checkout flow, for example validating the items are in stock
or that the customer is allowed to purchase the items. This callback is synchronous and has a timeout of 10 seconds.
It differs from the notificationUri callback that is asynchronous and mainly used to notify you about completed purchases.
Property | Required | Explanation |
---|---|---|
callbackUri | Yes | The endpoint to be called whenever any of the callback types occurs. Must accept POST requests. Use HTTPS for security reasons. |
callbackTypes | Yes | Currently ValidateOrder and PaymentFailed are supported. |
The request body of the callback contains the callback type, along with the private id and public token. Use the private id to validate that the callback originates from Walley
// Example of request body for a callback
{
"type": "ValidateOrder",
"body": {
"checkoutId": "3350f4f2-809e-4103-a566-85e20280b76a",
"publicToken": "public-SE-0a94436dba53edc468584935e7da524eb8d5d8feab85ed03"
}
}
ValidateOrder
This callback will be called just before payment, in order to validate the purchase. Read more
PaymentFailed
This callback will be called if a payment was unsuccessful, for instance if there was no money on the card or the user cancelled electronic verification. You can use this callback to undo any logic on your side that you made in the ValidateOrder
callback.
Exceptions for when we will not be sending this callback are:
- If payment fails before the
ValidateOrder
callback. - If you respond with anything other than 2XX during the
ValidateOrder
callback.
These callbacks have a timeout of 10 seconds. Right now, the Checkout doesn't use the callback's response for anything.
Cart object
The cart
object contains an array of items with the following properties. Please note that at least one article has to be included in order to initialize a checkout session.
Property | Required | Explanation |
---|---|---|
id | Yes | The article id or equivalent. Max 50 characters. Values are trimmed from leading and trailing white-spaces. Shown on the invoice or receipt. |
description | Yes | Descriptions longer than 50 characters will be truncated. Values are trimmed from leading and trailing white-spaces. Shown on the invoice or receipt. |
type | No | An optional type of the article. Currently the only valid value is GiftCard . If specified, unitPrice must be negative, quantity must be 1, vat must be 0.0. Furthermore unitWeight , requiresElectronicId , minimumAge and maximumAge cannot be specified. Read more about Gift Cards |
unitPrice | Yes | The unit price of the article including VAT. Both positive and negative values allowed. Max 2 decimals, i.e. 100.00 |
unitWeight | No | The weight of the article. Only positive values are allowed (including zero) |
quantity | Yes | The quantity of the article. Allowed values are 1 to 99999999 . |
vat | Yes | The VAT of the article in percent. Allowed values are 0 to 100 . Max 2 decimals, i.e. 25.00 |
requiresElectronicId | No | To minimize the risk of a fraudulent purchase on credit products, it is possible to force the customer to strongly identify themselves at the point of purchase using electronic id such as Mobilt BankID. An example would be selling tickets that are delivered electronically. This feature does not validate the age of the customer and is supported for credit payments for B2C and B2B on the Swedish, Norwegian and Finnish markets. |
minimumAge | No | The minimum age allowed to purchase this product. Allowed values are 1 to 120 . When this property is set, we require the user to enter a civic number and verify it with electronic id before making the payment. If multiple items has different minimumAge, we use the oldest age. This feature is supported for B2C. |
maximumAge | No | The maximum age allowed to purchase this product. Allowed values are 1 to 120 . When this property is set, we require the user to enter a civic number and verify it with electronic id before making the payment. If multiple items has different maximumAge, we use the youngest age. This feature is supported for B2C. |
sku | No | A stock Keeping Unit is a unique alphanumeric code that is used to identify product types and variations. Maximum allowed characters are 1024. |
The shippingProperties
are optional and only relevant if a Delivery Module is used.
It gives flexibility to customize what delivery methods should be available based on the items in the cart.
Read more about how these properties are included when Fetching Delivery Methods with nShift, or Fetching Delivery Methods with Custom Delivery Adapter.
Fees object (optional)
The Fees
object allows you to set a shipping fee.
The fee will be shown last on the receipt after all the cart items and also on the purchase confirmation page for a completed purchase.
This object is optional and can be set after initialization of the checkout through the Update fees endpoint. Note that if you are using Custom Delivery Adapter that the shipping fee is taken care of there instead.
Property | Required | Explanation |
---|---|---|
id | Yes | An id of the fee item. Max 50 characters. Values are trimmed from leading and trailing white-spaces. Shown on the invoice or receipt. |
description | Yes | Descriptions longer than 50 characters will be truncated. Values are trimmed from leading and trailing white-spaces. Shown on the invoice or receipt. |
unitPrice | Yes | The unit price of the fee including VAT. Allowed values are 0 to 999999.99 . Max 2 decimals, i.e. 25.00 |
vat | Yes | The VAT of the fee in percent. Allowed values are 0 to 100 . Max 2 decimals, i.e. 25.00 |
PrivateCustomerPrefill object (optional)
You can use the privateCustomerPrefill
object to share any information you have on the customer. We use this data to try and identify the customer, making their checkout faster. If we can't identify them, we'll ask the customer for more details.
However, whether we identify the customer or not doesn't change the response or status code of the Initialize Checkout Session call.
We recommend providing complete data when using prefill.
Examples
- If
email
andmobilePhoneNumber
are provided and the combination is matched for a previously known customer, the user will be logged in and ready for purchase. - If
email
andmobilePhoneNumber
are provided but the combination does not match a previously known customer, we will prompt the user for further identification details. - If
email
andmobilePhoneNumber
are provided together withnationalIdentificationNumber
we will try to identify the customer using these values by doing a lookup in an address registry. - If a complete
deliveryAddress
is provided the address will be set as the customer's delivery address. - If a non-complete
deliveryAddress
is provided the address will be shown upon initial identification for the customer if the customer choses to not enter their national registration number.
Property | Required | Explanation |
---|---|---|
No * | The customer's email address. | |
mobilePhoneNumber | No * | The customer's mobile phone. When providing a number from a foreign country, it must have a valid prefix such as +46 or 0046. |
nationalIdentificationNumber | No | The customer's national identification number. |
deliveryAddress | No | Delivery Address (see below) |
*
email and mobilePhoneNumber are required when identifying a user using a profile with DeliveryMode: Digital
DeliveryAddress object (B2C)
Property | Required | Explanation |
---|---|---|
firstName | No | First name |
lastName | No | Last name |
coAddress | No | Care of address |
address | No | Primary address row |
address2 | No | Additional address row |
postalCode | No | Postal code |
city | No | City |
countryCode | No | Only used when shipping to multiple countries |
Prefill or Preset of a business customer
You can use prefill and/or preset data to set up a business customer's details before showing the checkout page. Prefill information is like a suggestion; customers can change it. However, preset information can't be changed by the customer, so you must ensure it's correct. The prefill and preset objects are equal in structure and when the checkout combines the information from the two objects, the preset values are prioritized over the prefilled values.
Though all object values are optional, there are validation rules enforced at initalization.
If the combined object includes an organization number, you must also provide a full buyer's object to identify the customer. If the organization number is preset and we can't identify the business (or the buyer's object is missing), we'll give an error. However, if the organization number is prefilled and we can't identify, the customer will have to do the identification themselves.
If the values marked with a *
in the DeliveryAddress object are provided, we'll set the customer's delivery address after identifying them. If any of these values are preset, the customer won't be able to change the delivery address later.
Property | Required | Explanation |
---|---|---|
organizationNumber | No | The organization number of the company |
buyer | No | Buyer information (see below) |
invoiceReference | No | Shown on the invoice |
invoiceTag | No | Shown on the invoice |
deliveryAddress | No | Delivery Address (see below) |
Buyer object
A buyer is the person performing a purchase on the behalf of the company
Property | Required | Explanation |
---|---|---|
firstName | No | The first name of the buyer |
lastName | No | The last name of the buyer |
No | The buyer's email address. | |
mobilePhoneNumber | No | The buyer's mobile phone. When providing a number from a foreign country, it must have a valid prefix such as +46 or 0046. |
DeliveryAddress object (B2B)
Property | Required | Explanation |
---|---|---|
companyName | No * | Name of the company |
coAddress | No | Care of address |
address | No * | Primary address row |
address2 | No | Additional address row |
postalCode | No * | Postal code |
city | No * | City |
If you provide any of the values marked with *
, you must provide all of them in order to have a valid address. The exception is for Norway where address
is optional.
CustomFields (optional)
The customFields
array allows you to optionally provide a blueprint for fields of your choosing to be rendered inside the checkout. These can be things like consent for newsletter or comments.
It will be rendered in it's own section after the payment methods.
You can specify multiple groups that will be rendered as their own separate sections, and the name
field will set the header. We recommend not to have a name present if you only have one group.
Each group can contain fields. We currently support checkboxes (shown as animated switches) and text fields. They may have a default value.
Every group and field can also contain overriding localization information for markets with multiple languages, or to simplify with one configuration regardless of the current session language.
Every level also supports a metadata object (see below for more information).
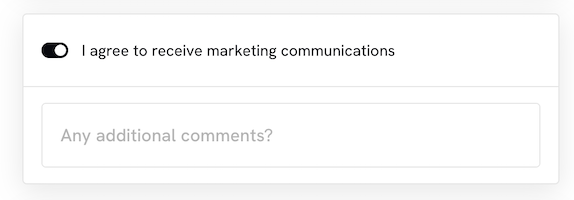
Group object
Property | Required | Explanation |
---|---|---|
id | Yes | An id for the group. Max 50 characters. Values are trimmed from leading and trailing white-spaces. |
name | No | The header rendered above the group section |
metadata | No | A metadata object for the group (see below for more info) |
localizations | No | Specify a different name and metadata for other languages (specified with ISO language codes) |
fields | Yes | An array of fields to render (see below) |
Fields object
Property | Required | Explanation |
---|---|---|
id | Yes | An id for the field. Max 50 characters. Values are trimmed from leading and trailing white-spaces. |
name | Yes | The label for the field |
type | Yes | The type of field to be rendered. "Checkbox" or "Text" |
value | No | The default value for the field. A string for text fields and true/false for checkboxes |
metadata | No | A metadata object for the group (see below for more info) |
localizations | No | Specify a different name/value/metadata for other languages (specified with ISO language codes) |
Metadata object
The metadata
object allows you to optionally provide custom metadata information in a key/value format of at most 6000 characters. Metadata is not processed by Walley but will be returned when retrieving information about the checkout. This means that the metadata
object can be used as an opaque reference for things that needs to be remembered without saving state locally.
Each metadata key must be a string but the value can be any valid JSON type.
Response
Property | Explanation |
---|---|
publicToken | The publicToken is used to render the Checkout iframe. The public token has a limited lifetime of 168 hours (7 days). |
privateId | The privateId is used to perform backend communication with the Checkout API for the given session. The privateId is used to acquire information for an ongoing or completed session for up to 180 days after it was created. The privateId is also used when modifying a session (e.g. cart update). Updates can be made for a session until it is completed or expiresAt has passed. |
expiresAt | The timestamp when this Checkout session will expire. After this timestamp the purchase cannot be completed, no updates to the cart can be performed and a new Checkout session must be initialized. |
paymentUri | A shortcut link to this Checkout session. Used when distributing a Pay Link to a customer. |
paymentQrCodeUri | A QR code representation of paymentUri . The URL returns an image that can be embedded in the merchant's system. Note that this URL is publicly available so no auth is required. The URL supports the same URL parameters as paymentUri . |
Templating the uri values
The notificationUri
, validationUri
, redirectPageUri
and the hostedPaymentPageAbortedRedirectUriUri
properties can optionally be templated.
A correctly templated specified uri will replace the varibles with the real values for the given Checkout session when called.
Allowed template values:
Uri | {checkout.publictoken} | {checkout.id} |
---|---|---|
notificationUri | ✔️ | ✔️ |
validationUri | ✔️ | ✔️ |
redirectPageUri | ✔️ | ❌ |
hostedPaymentPageAbortedRedirectUri | ✔️ | ❌ |
The variable names {checkout.id}
will be replaced by the privateId
and {checkout.publictoken}
will be replaced by publicToken
. Note that the variables are case-sensitive.